Tip Tuesday | Python Data Type Hints
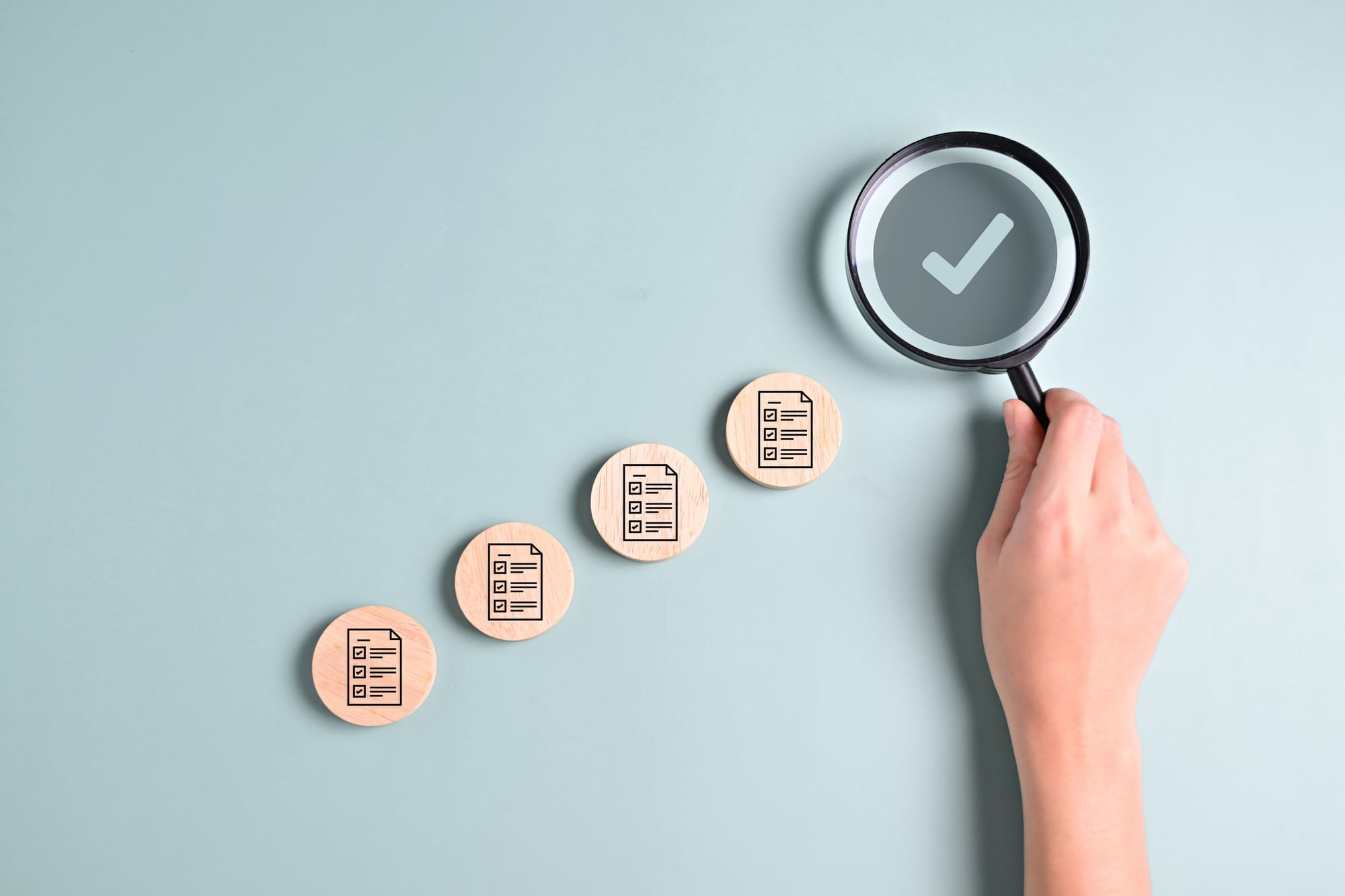
Data type hints in Python, introduced in PEP 484 and enhanced in subsequent PEPs, provide a way to specify the expected types of variables, function parameters, and return values. While Python remains dynamically typed, type hints allow developers to add optional static typing to their code for documentation, readability, and tooling support. Here’s an overview of how data type hints work in Python:
- Type Hinting for Variables:
# Specify the expected type of a variable
name: str = "Jon"
age: int = 30
price: float = 12.5
is_valid: bool = True
- Type Annotations for Function Parameters and Return Types:
def greet(name: str) -> str:
return f"Hello, {name}"
def add(a: int, b: int) -> int:
return a + b
- Type Hinting for Class Attributes:
class Person:
def __init__(self, name: str, age: int) -> None:
self.name: str = name
self.age: int = age self.age: int = age
- Type Hinting for Lists, Tuples, and Dictionaries (Composite types):
Type hints can also specify composite types like lists, tuples, dictionaries, and sets.
from typing import List, Tuple, Dict
# List of integers
numbers: List[int] = [1, 2, 3]
# Tuple of string and integer
person: Tuple[str, int] = ("Alice", 25)
# Dictionary with string keys and integer values
scores: Dict[str, int] = {"Math": 90, "Science": 85}
- Using Optional and Union for Nullable and Multiple Types:
from typing import Optional, Union
# Nullable integer
maybe_age: Optional[int] = None
# Union of integer and float
result: Union[int, float] = 10
- Type Aliases:
You can create aliases for complex types to improve readability.
from typing import List
Vector = List[float]
def scale(scalar: float, vector: Vector) -> Vector:
return [scalar * num for num in vector]
- Avoiding Unnecessary Type Hints: While type hints are beneficial, avoid adding them where the type is obvious from context or when they don’t add clarity. Python’s dynamic nature means that not every variable requires a type hint.
- Using Static Analysis Tools: Utilise tools like mypy to perform static type checking. These tools can help identify potential type-related errors in your code, enhancing its robustness.
By adopting these practices, you can harness the power of type hints to improve code quality, readability, and maintainability in your Python projects.